使用BeautifulSoup进行网页抓取
今天,您将了解如何使用 BeautifulSoup 进行网页抓取。您将学习如何使用 requests 库来获取网页以及如何使用BeautifulSoup进行网页抓取来解析Python中的HTML。
在本教程中,您将学习:
- 网页抓取和解析的基础知识
- 如何安装BeautifulSoup
- 如何解析本地HTML文件
- 使用Requests和BeautifulSoup来抓取和解析网页
- 在 HTML 中查找元素所需的方法
- 可用于改进 HTML 内部匹配的过滤器
什么是BeautifulSoup
BeautifulSoup是 Python 中的一个解析库,用于从 HTML 或 XML 中抓取信息。BeautifulSoup 解析器提供了 Python 习惯用法来搜索和修改解析树。
简而言之,BeautifulSoup 是一个库,允许您以可用的方式格式化 HTML 并从中提取元素。
网络抓取是使用机器人从网站提取数据并将其导出为易于理解的格式的过程。网络抓取工具从网页中提取 HTML 代码,然后对其进行解析以提取有价值的信息。
BeautifulSoup 是一个 Python 库,可以轻松BeautifulSoup解析HTML 或 XML 以从中提取有价值的信息。
网络抓取中的解析是将非结构化数据转换为更易于读取、使用和提取数据的结构化格式(例如解析树)的过程。基本上,解析意味着将文档分割成可用的块。
推荐:使用BeautifulSoup查找具有给定属性值的标签
BeautifulSoup 入门
在Python中使用pip安装BeautifulSoup。
$ pip install beautifulsoup4
使用 BeautifulSoup 进行简单解析,这是一个使用BeautifulSoup解析HTML解析器的简单示例:
from bs4 import BeautifulSoup
soup = BeautifulSoup("<p>I am learning <span>BeautifulSoup</span></p>")
soup.find('span')
<span>BeautifulSoup</span>
如何使用 BeautifulSoup 解析 HTML
要解析的 HTML 示例
html = '''
<html>
<head>
<title>Simple SEO Title</title>
<meta name="description" content="Meta Description with less than 300 characters.">
<meta name="robots" content="noindex, nofollow">
<link rel="alternate" href="https://www.example.com/en" hreflang="en-ca">
<link rel="canonical" href="https://www.example.com/fr">
</head>
<body>
<header>
<div class="nav">
<ul>
<li class="home"><a href="#">Home</a></li>
<li class="blog"><a class="active" href="#">Blog</a></li>
<li class="about"><a href="#">About</a></li>
<li class="contact"><a href="#">Contact</a></li>
</ul>
</div>
</header>
<div class="body">
<h1>Blog</h1>
<p>Lorem ipsum dolor <a href="#">Anchor Text Link</a> sit amet consectetur adipisicing elit. Ipsum vel laudantium a voluptas labore. Dolorum modi doloremque, dolore molestias quos nam a laboriosam neque asperiores fugit sed aut optio earum!</p>
<h2>Subtitle</h2>
<p>Lorem ipsum dolor sit amet consectetur adipisicing elit. Ipsum vel laudantium a voluptas labore. Dolorum modi doloremque, dolore molestias quos nam a <a href="#" rel="nofollow">Nofollow link</a> laboriosam neque asperiores fugit sed aut optio earum!</p>
</div>
</body>
</html>'''
我们刚刚创建的 HTML 变量类似于我们抓取网页时得到的输出。这是 HTML,但存储为文本。可以使用正则表达式来解析文本内容,但有更好的方法:使用BeautifulSoup解析HTML进行解析。
解析 HTML
要使用 BeautifulSoup解析HTML,请通过添加要解析的 HTML 作为必需参数并将解析器的名称作为可选参数来实例化 BeautifulSoup 构造函数。
# Parsing an HTML File
from bs4 import BeautifulSoup
import requests
soup = BeautifulSoup(html, 'html.parser')
这将返回解析后的 HTML 并根据 HTML 响应创建一个 BeautifulSoup 对象。
然后,我们使用该方法从 BeautifulSoup 对象中检索任何 HTML 元素(在本例中为标题标签) soup.find()
。
print(soup.find('title'))
<title>Simple SEO Title</title>
使用 BeautifulSoup 解析本地 HTML 文件
如果您的计算机上保存有 HTML 文件,您还可以使用BeautifulSoup解析HTML解析本地 HTML 文件。
# Parsing an HTML File
from bs4 import BeautifulSoup
import requests
with open('/path/to/file.html') as f:
soup = BeautifulSoup(f, 'html.parser')
print(soup)
要使用 BeautifulSoup 解析网页,请使用Python requests 库获取页面的 HTML 。您可以通过多种不同的方式访问页面的 HTML:HTTP 请求、基于浏览器的应用程序、从 Web 浏览器手动下载。
在Crawler-test.com上练习网页抓取
在本教程中,我们将在为此目的创建的玩具网站上使用 BeautifulSoup 练习网页抓取:crawler-test.com。
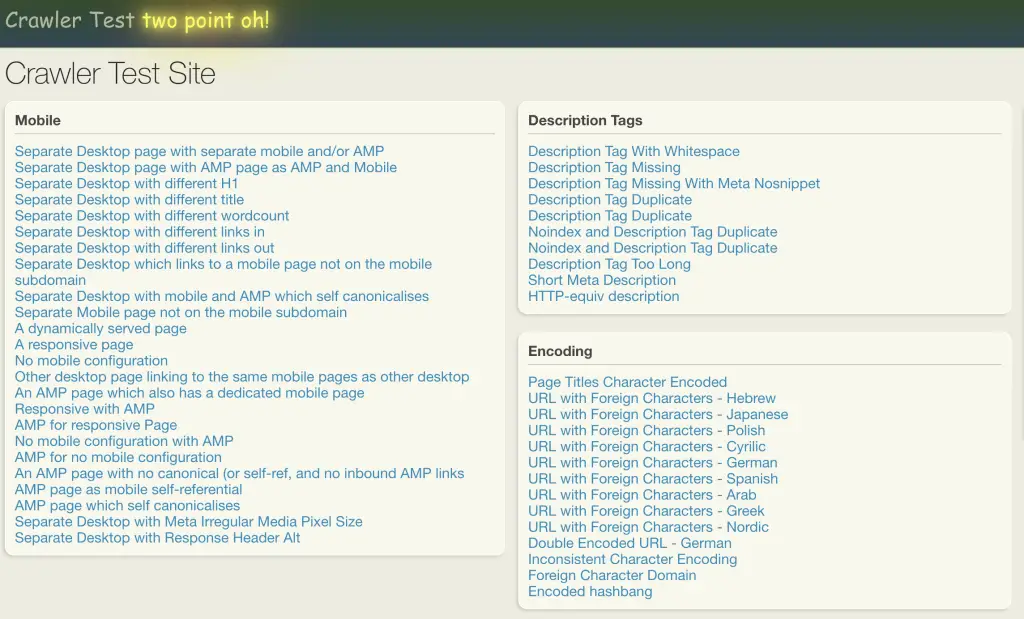
使用请求从网页中提取内容
要从网页中提取内容,请使用 Python requests 库向 URL 发出 HTTP 请求。
# Making an HTTP Request
import requests
url = 'https://crawler-test.com/'
response = requests.get(url)
print('Status code: ', response.status_code)
print('Text: ', response.text[:50])
使用 BeautifulSoup 解析响应
要使用 BeautifulSoup 解析响应,请将检索到的 HTML 添加为 BeautifulSoup 构造函数的参数。当获取带有请求的网页时,将返回响应对象。从该响应中,您可以检索页面的 HTML。HTML 以 Unicode 或字节(文本)形式存储。
我们已经了解了如何使用 BeautifulSoup解析HTML 的文本表示。为此,我们需要将response.text 传递给BeautifulSoup 类。
from bs4 import BeautifulSoup
# Parse the HTML
soup = BeautifulSoup(response.text, 'html.parser')
# Extract any HTML tag
soup.find('title')
<title>Crawler Test Site</title>
如何使用 BeautifulSoup 提取 HTML 标签
使用 BeautifulSoupfind()
和find_all()
方法从解析的 HTML 中提取 HTML 标签。
您需要抓取的一些非常常见的 HTML 标签是标题、h1 和链接。
按标签名称查找元素
要在 BeautifulSoup 中通过标签名称查找 HTML 元素,请将标签名称作为参数传递给 BeautifulSoup 对象的方法。
以下是如何使用 bs4 提取这些标签的示例。
# Extracting HTML tags
title = soup.find('title')
h1 = soup.find('h1')
links = soup.find_all('a', href=True)
# Print the outputs
print('Title: ', title)
print('h1: ', h1)
print('Example link: ', links[1]['href'])
Title: <title>Crawler Test Site</title>
h1: <h1>Crawler Test Site</h1>
Example link: /mobile/separate_desktop
按 ID 查找元素
要在 BeautifulSoup 中通过 ID 查找 HTML 元素,请将 id 名称传递给id
soup 对象的 find() 方法的参数。
soup.find(id="header")
<div id="header">
<a href="/" id="logo">Crawler Test <span class="neon-effect">two point oh!</span></a>
<div style="position:absolute;right:520px;top:-4px;"></div>
</div>
按 HTML 类名称查找元素
要在BeautifulSoup解析HTML中按其类查找 HTML 元素,请将字典作为 soup 对象方法的参数传递find_all()
。
soup.find_all('div', {'class': 'panel-header'})[0]
<div class="panel-header">
然后您可以循环该对象来执行您想要的任何操作。
# Find Elements by Class
boxes = soup.find_all('div', {'class': 'panel'})
box_names = []
for box in boxes:
title = box.find('h3')
box_names.append(title.text)
box_names[:5]
['Mobile', 'Description Tags', 'Encoding', 'Titles', 'Robots Protocol']
使用 CSS 选择器选择元素
按类名选择元素的替代方法是使用select()
BeautifulSoup 对象上的方法并使用CSS 选择器来查看内容。
soup.select('div .panel-header')
如何从 HTML 元素中提取文本
要使用BeautifulSoup解析HTML从 HTML 元素中提取文本,请使用.text
soup 对象上的属性。如果对象是一个列表(例如使用 找到find_all
),请使用 for 循环迭代每个元素并在每个元素上使用 text 属性。
logo = soup.find('a', {'id': 'logo'})
logo.text
'Crawler Test two point oh!'
如何在 Bs4 中提取带有属性的 HTML 标签
要使用BeautifulSoup解析HTML标签的属性提取 HTML 标签,请将字典传递给attrs
find() 方法中的参数。
示例属性是name
在元描述中使用的属性,或者href
在超链接中使用的属性。
某些 HTML 元素要求您使用其属性来获取元素。
下面,我们将解析元描述和元机器人名称属性。
description = soup.find('meta', attrs={'name':'description'})
meta_robots = soup.find('meta', attrs={'name':'robots'})
print('description: ',description)
print('meta robots: ',meta_robots)
description: <meta content="Default description XIbwNE7SSUJciq0/Jyty" name="description"/>
meta robots: None
在这里,元机器人元素不可用,因此返回None
。
解析不可用标签
下面,我们将从网页中抓取描述、元机器人和规范标签。如果它们不可用,我们将返回一个空字符串。
# Conditional parsing
canonical = soup.find('link', {'rel': 'canonical'})
# Extract if attribute ifound
description = description['content'] if description else ''
meta_robots = meta_robots['content'] if meta_robots else ''
canonical = canonical['href'] if canonical else ''
# Print output
print('description: ', description)
print('meta_robots: ', meta_robots)
print('canonical: ', canonical)
description: Default description XIbwNE7SSUJciq0/Jyty
meta_robots:
canonical:
如何提取网页上的所有链接
要使用BeautifulSoup解析HTML提取网页的所有链接,请使用soup.find_all()
以"a"
标签作为参数的方法。将href
参数设置为 True。
soup.find_all('a', href=True)
在网页抓取中提取网页上的链接是最相关的事情。
现在,我们将学习如何做到这一点。
此外,我们将学习如何使用 urllib 中的 urljoin 方法来克服构建网络爬虫最常见的挑战之一:处理相对和绝对 URL。
from urllib.parse import urlparse, urljoin
url = 'https://crawler-test.com/'
# Parse URL
parsed_url = urlparse(url)
domain = parsed_url.scheme + '://' + parsed_url.netloc
print('Domain root is: ', domain)
# Get href from all links
links = []
for link in soup.find_all('a', href=True):
# join domain to path
full_url = urljoin(domain, link['href'])
links.append(full_url)
# Print output
print('Top 5 links are :\n', links[:5])
Domain root is: https://crawler-test.com
Top 5 links are :
['https://crawler-test.com/', 'https://crawler-test.com/mobile/separate_desktop', 'https://crawler-test.com/mobile/desktop_with_AMP_as_mobile', 'https://crawler-test.com/mobile/separate_desktop_with_different_h1', 'https://crawler-test.com/mobile/separate_desktop_with_different_title']
提取页面特定部分的元素
要提取页面特定部分上的元素(例如使用其 id),请将 soup.find() 的结果分配给另一个变量,并在该对象上使用 bs4 内置方法之一。
# Get div that contains a specific ID
subset = soup.find('div', {'id': 'idname'})
# Find all p inside that div
subset.find_all('p')
例子:
# Extract all links on the page
from urllib.parse import urljoin
domain = 'https://crawler-test.com/'
# Get div that contains a specific ID
menu = soup.find('div', {'id': 'header'})
# Find all links within the div
menu_links = menu.find_all('a', href=True)
# Print output
for link in menu_links[:5]:
print(link['href'])
print(urljoin(domain, link['href']) )
/
https://crawler-test.com/
使用 BeautifulSoup 删除特定的 HTML 标签
要使用BeautifulSoup解析HTML从 HTML 文档中删除特定的 HTML 标签,请使用 方法decompose()
。
soup.tag_name.decompose()
例子
# Prompt
wikipedia_text = '''
<p>In the United States, website owners can use three major <a href="/wiki/Cause_of_action" title="Cause of action">legal claims</a> to prevent undesired web scraping: (1) copyright infringement (compilation), (2) violation of the <a href="/wiki/Computer_Fraud_and_Abuse_Act" title="Computer Fraud and Abuse Act">Computer Fraud and Abuse Act</a> ("CFAA"), and (3) <a href="/wiki/Trespass_to_chattels" title="Trespass to chattels">trespass to chattel</a>.<sup id="cite_ref-6" class="reference"><a href="#cite_note-6">[6]</a></sup> However, the effectiveness of these claims relies upon meeting various criteria...</p>'''
# Parse HTML
wiki_soup = BeautifulSoup(wikipedia_text, 'html.parser')
# Get first paragraph
par = wiki_soup.find_all('p')[0]
# Get all links
par.find_all('a')
par.sup.decompose()
par.find_all('a')
按文本内容查找元素
string
要使用文本内容查找 HTML 中的元素,请将要匹配的文本添加为方法内的参数值find_all()
。
# Getting Elements using Text
soup = BeautifulSoup(r.text, 'html.parser')
soup.find_all('a',string="Description Tag Too Long")
[<a href="/description_tags/description_over_max">Description Tag Too Long</a>]
这样做的问题是字符串必须完全匹配。因此,使用部分字符串与 BeautifulSoup 匹配不会返回任何内容:
soup.find_all('a',string="Description")
解决此字符串匹配问题的方法是使用 apply 函数或将正则表达式与string
参数一起使用。接下来我们将介绍这两种情况。
将函数应用于 BeautifulSoup 方法
要在 BeautifulSoup 方法内部应用函数,请将该函数添加到该find_all()
方法的字符串参数中。
def find_a_string(value):
return lambda text: value in text
soup.find_all(string=find_a_string('Description Tag'))
['Description Tags',
'Description Tag With Whitespace',
'Description Tag Missing',
'Description Tag Missing With Meta Nosnippet',
'Description Tag Duplicate',
'Description Tag Duplicate',
'Noindex and Description Tag Duplicate',
'Noindex and Description Tag Duplicate',
'Description Tag Too Long']
在 BeautifulSoup 中使用正则表达式解析 HTML 页面
要使用正则表达式通过BeautifulSoup解析HTML页面,请导入re
模块,并将re.compile()
对象分配给方法的字符串参数find_all()
。
from bs4 import BeautifulSoup
import re
# Parse using regex
soup = BeautifulSoup(r.text, 'html.parser')
soup.find_all(string=re.compile('Description Tag'))
['Description Tags',
'Description Tag With Whitespace',
'Description Tag Missing',
'Description Tag Missing With Meta Nosnippet',
'Description Tag Duplicate',
'Description Tag Duplicate',
'Noindex and Description Tag Duplicate',
'Noindex and Description Tag Duplicate',
'Description Tag Too Long']
如何将 XPath 与 BeautifulSoup 结合使用 (lxml)
要使用 XPath 通过BeautifulSoup解析HTML文档中提取元素,您需要安装 lxml python 库,因为 Beautiful 不支持XPath 表达式。
from lxml import html
# Parse HTML with XPath
content = html.fromstring(r.content)
panels = content.xpath('//*[@class="panel-header"]')
# get text from tags
[panel.find('h3').text for panel in panels][:5]
查找 HTML 元素的父元素、子元素和兄弟元素
BeautifulSoup 返回一个解析树,您可以在树中的每个父级、子级和同级中移动以查找所需的元素。
查找 HTML 元素的父元素
要查找 HTML 元素的单父元素,请使用该find_parent()
方法,该方法将显示您正在查找的元素及其在BeautifulSoup解析HTML树中的父元素。
a_child = soup.find_all('a')[0]
a_child.find_parent()
<div id="header">
<a href="/" id="logo">Crawler Test <span class="neon-effect">two point oh!</span></a>
<div style="position:absolute;right:520px;top:-4px;"></div>
</div>
要查找 HTML 元素的所有父元素,请使用find_parents()
BeautifulSoup 对象上的方法。
a_child.find_parents()
查找 HTML 元素的子元素
要查找 HTML 元素的单个子元素,请使用findChild()
将在 HTML 树中显示该元素的子元素的方法。
a_child = soup.find_all('a')[0]
a_child.findChild()
<span class="neon-effect">two point oh!</span>
要查找 HTML 元素的所有子元素,请使用fetchChildren()
BeautifulSoup 对象上的方法。
a_child.findChildren()
#or
list(a_child.children)
查找 HTML 元素的所有后代。
list(a_child.descendants)
查找 HTML 元素的同级元素
要查找 HTML 元素之后的下一个同级元素,请使用find_next_sibling()
将在 HTML 树中显示下一个同级元素的方法。
a_child = soup.find_all('a')[0]
a_child.find_next_sibling()
a_child.find_next_siblings()
<div style="position:absolute;right:520px;top:-4px;"></div>
这是从哪里来的,如果你采用父元素:
a_parent = soup.find('div',{'id':'header'})
a_parent
您获得该元素的 HTML,并获得 <a> 标记的下一个同级,即突出显示的 div(以及之前显示的结果。
<div id="header">
<a href="/" id="logo">Crawler Test <span class="neon-effect">two point oh!</span></a>
<div style="position:absolute;right:520px;top:-4px;"></div>
</div>
同样,您也可以找到以前的兄弟姐妹。
a_child. find_previous_sibling()
a_child. find_previous_siblings()
要查找 HTML 元素的所有父元素,请使用find_parents()
BeautifulSoup 对象上的方法。
使用 BeautifulSoup 修复损坏的 HTML
prettify()
使用 BeautifulSoup,您可以使用BeautifulSoup 对象上的方法获取损坏的 HTML 并完成缺失的部分。
from bs4 import BeautifulSoup
soup = BeautifulSoup("<p>Incomplete Broken <span>HTML<</p>")
print(soup.prettify())
<html>
<body>
<p>
Incomplete Broken
<span>
HTML
</span>
</p>
</body>
</html>
如何使用 urllib 和 BeautifulSoup 在 Python 中抓取网页
Urllib 可以与 Bs4 结合使用,作为 Python requests 库的替代方案,在 Python 中从 Web 检索信息。
要使用 urllib 和 BeautifulSoup 抓取网页,请使用 urllib.request 中的 urlopen() 方法,并将解码后的响应传递给 BeautifulSoup 类。
from bs4 import BeautifulSoup
from urllib.request import urlopen
r = urlopen('https://www.crawler-test.com')
html = r.read().decode("utf8")
soup = BeautifulSoup(html)
soup.find('title')
如何将 BeautifulSoup 与 Requests-HTML 结合使用
BeautifulSoup 可以解析您提供的任何 HTML,从而可以解析Requests-HTML响应。要使用 BeautifulSoup 解析 Requests-HTML 对象的 HTML,请将 response.html.raw_html 属性传递给 BeautifulSoup 对象。
# requests-html beautifulsoup
from bs4 import BeautifulSoup
from requests_html import HTMLSession
url = 'https://crawler-test.com/'
session = HTMLSession()
r = session.get(url)
soup = BeautifulSoup(r.html.raw_html, features='lxml')
soup.find('h1')
在 Python 中将 BeautifulSoup 的输出写入 HTML 文件
使用 Python BeautifulSoup 的 open() 函数将输出写入 HTML 文件。此外,使用 BeautifulSoup 对象的 prettify() 方法可以修复潜在的错误并生成更漂亮的输出。
# write the output to html file with BeautifulSoup
with open('filename.html', 'w') as f:
pretty_soup = str(soup.prettify())
f.write(pretty_soup)
BeautifulSoup方法
当列出 BeautifulSoup 方法时,您会发现方法名称以两种不同的大小写形式编写:camelCase 和 Snake_case。BeautifulSoup之前的版本使用Camel case,最新版本使用snake_case。
BeautifulSoup方法列表
Description | 描述 |
append() | 将给定的 PageElement 附加到该 PageElement 的内容中。 |
childGenerator() | 已弃用的生成器。 |
clear() | 通过调用 extract() 来清除此 PageElement 的所有子元素。 |
currentTag() | 表示已解析的 HTML 或 XML 文档的数据结构。 |
decode() | 将解析树的字符串或 Unicode 表示形式返回为 HTML 或 XML 文档。 |
decode_contents() | 将此标签的内容呈现为 Unicode 字符串。 |
decompose() | 递归地销毁此 PageElement 及其子元素。 |
encode() | 呈现此 PageElement 及其内容的字节字符串表示形式。 |
encode_contents() | 将此 PageElement 的内容呈现为字节串。 |
endData() | 当数据段结束时 TreeBuilder 调用的方法。 |
extend() | 将给定的 PageElements 附加到该元素的内容中。 |
extract() | 破坏性地将这个元素从树上撕下来。 |
fetchNextSiblings() | 查找此 PageElement 的所有与给定条件匹配并稍后出现在文档中的同级元素。 |
fetchParents() | 查找此 PageElement 的所有符合给定条件的父级。 |
fetchPrevious() | 从该 PageElement 开始向后查找文档,并查找与给定条件匹配的所有 PageElement。 |
fetchPreviousSiblings() | 返回此 PageElement 中符合给定条件并出现在文档前面的所有同级元素。 |
find() | 查看此 PageElement 的子元素并找到第一个与给定条件匹配的 PageElement。 |
findAll() | 查看此 PageElement 的子元素并查找与给定条件匹配的所有 PageElement。 |
findAllNext() | 查找与给定条件匹配且在文档中出现得晚于此 PageElement 的所有 PageElement。 |
findAllPrevious() | 从该 PageElement 开始向后查找文档,并查找与给定条件匹配的所有 PageElement。 |
findChild() | 查看此 PageElement 的子元素并找到第一个与给定条件匹配的 PageElement。 |
findChildren() | 查看此 PageElement 的子元素并查找与给定条件匹配的所有 PageElement。 |
findNext() | 查找第一个与给定条件匹配且在文档中出现得晚于此 PageElement 的 PageElement。 |
findNextSibling() | 查找与此 PageElement 最接近的、与给定条件匹配并随后出现在文档中的同级元素。 |
findNextSiblings() | 查找此 PageElement 的所有与给定条件匹配并稍后出现在文档中的同级元素。 |
findParent() | 查找此 PageElement 与给定条件匹配的最接近的父级。 |
findParents() | 查找此 PageElement 的所有符合给定条件的父级。 |
findPrevious() | 从该 PageElement 开始向后查找文档,找到第一个与给定条件匹配的 PageElement。 |
findPreviousSibling() | 返回与此 PageElement 最接近的、与给定条件匹配并出现在文档中较早位置的同级元素。 |
findPreviousSiblings() | 返回此 PageElement 中符合给定条件并出现在文档前面的所有同级元素。 |
find_all() | 查看此 PageElement 的子元素并查找与给定条件匹配的所有 PageElement。 |
find_all_next() | 查找与给定条件匹配且在文档中出现得晚于此 PageElement 的所有 PageElement。 |
find_all_previous() | 从该 PageElement 开始向后查找文档,并查找与给定条件匹配的所有 PageElement。 |
find_next() | 查找第一个与给定条件匹配且在文档中出现得晚于此 PageElement 的 PageElement。 |
find_next_sibling() | 查找与此 PageElement 最接近的、与给定条件匹配并随后出现在文档中的同级元素。 |
find_next_siblings() | 查找此 PageElement 的所有与给定条件匹配并稍后出现在文档中的同级元素。 |
find_parent() | 查找此 PageElement 与给定条件匹配的最接近的父级。 |
find_parents() | 查找此 PageElement 的所有符合给定条件的父级。 |
find_previous() | 从该 PageElement 开始向后查找文档,找到第一个与给定条件匹配的 PageElement。 |
find_previous_sibling() | 返回与此 PageElement 最接近的、与给定条件匹配并出现在文档中较早位置的同级元素。 |
find_previous_siblings() | 返回此 PageElement 中符合给定条件并出现在文档前面的所有同级元素。 |
format_string() | 使用给定的格式化程序格式化给定的字符串。 |
formatter_for_name() | 如有必要,查找或创建给定标识符的格式化程序。 |
get() | 返回标签的“key”属性的值,如果没有该属性,则返回为“default”指定的值。 |
getText() | 获取此 PageElement 的所有子字符串,并使用给定的分隔符连接起来。 |
get_attribute_list() | 与 get() 相同,但始终返回一个列表。 |
get_text() | 获取此 PageElement 的所有子字符串,并使用给定的分隔符连接起来。 |
handle_data() | 当遇到文本数据块时由树构建器调用。 |
handle_endtag() | 当遇到结束标记时由树构建器调用。 |
handle_starttag() | 当遇到新标签时由树构建器调用。 |
has_attr() | 此 PageElement 是否具有具有给定名称的属性? |
has_key() | 已弃用的方法。这有点误导,因为 has_key() (属性)与 __in__ (内容)不同。 |
index() | 通过身份而不是值查找子项的索引。 |
insert() | 在此 PageElement 的子元素列表中插入一个新的 PageElement。 |
insert_after() | 此方法是 PageElement API的一部分,但 `BeautifulSoup` 没有实现它,因为在解析树中它之前或之后没有任何内容。 |
insert_before() | 此方法是 PageElement API 的一部分,但 `BeautifulSoup` 没有实现它,因为在解析树中它之前或之后没有任何内容。 |
new_string() | 创建一个与此 BeautifulSoup 对象关联的新 NavigableString。 |
new_tag() | 创建一个与此 BeautifulSoup 对象关联的新标签。 |
nextGenerator() | 生成器查找下一个元素 |
nextSiblingGenerator() | 生成器寻找下一个兄弟 |
object_was_parsed() | TreeBuilder 调用的方法将对象集成到解析树中。 |
parentGenerator() | 生成器寻找父级 |
parserClass() | 表示已解析的 HTML 或 XML 文档的数据结构。 |
parser_class() | 表示已解析的 HTML 或 XML 文档的数据结构。 |
popTag() | 关闭标签时由 _popToTag 调用的内部方法。 |
prettify() | 将此 PageElement 打印为字符串。 |
previousGenerator() | 生成器查找前一个元素 |
previousSiblingGenerator() | 生成器查找前一个兄弟姐妹 |
pushTag() | 打开标签时由handle_starttag调用的内部方法。 |
recursiveChildGenerator() | 已弃用的生成器。 |
renderContents() | 已弃用的 BS3 兼容性方法。 |
replaceWith() | 将此 PageElement 替换为一个或多个 PageElement,保持树的其余部分相同。 |
replaceWithChildren() | 将此 PageElement 替换为其内容。 |
replace_with() | 将此 PageElement 替换为一个或多个 PageElement,保持树的其余部分相同。 |
replace_with_children() | 将此 PageElement 替换为其内容。 |
reset() | 将此对象重置为某种状态,就好像它从未解析过任何标记一样。 |
select() | 对当前元素执行 CSS 选择操作。 |
select_one() | 对当前元素执行 CSS 选择操作。 |
setup() | 设置该元素与其他元素之间的初始关系。 |
smooth() | 通过合并连续的字符串来平滑该元素的子元素。 |
string_container() | |
unwrap() | 将此 PageElement 替换为其内容。 |
wrap() | 将此 PageElement 包裹在另一个 PageElement 中。 |
总结
以上是晓得博客为你介绍的使用BeautifulSoup进行网页抓取的全部内容,希望对你的BeautifulSoup学习有所帮助,了解BeautifulSoup解析HTML等内容,如有问题,可联系我们。