Python Lambda函数的10个实际用例
Lambda函数也称为匿名函数,是 Python 中的一项强大功能,允许您创建小型的一次性函数,而无需完整的函数定义。它们对于简单的操作特别有用,使您的代码更加简洁和可读。
在本文中,我们晓得博客将探讨Python Lambda函数的10个实际用例,展示它们在各种场景中的多功能性和实用性。
1、使用自定义键对列表进行排序
对列表进行排序时,Lambda 函数可用作自定义键函数,允许您根据特定属性或计算进行排序。
示例:根据学生的成绩对学生列表进行排序广告
students = [('Alice', 90), ('Bob', 85), ('Charlie', 92)]
sorted_students = sorted(students, key=lambda x: x[1])
print(sorted_students)
输出:
[('Bob', 85), ('Alice', 90), ('Charlie', 92)]
2、使用filter()函数过滤列表
filter() 函数可以与 lambda 函数结合使用,以根据特定条件过滤列表。
示例:从整数列表中过滤掉偶数广告
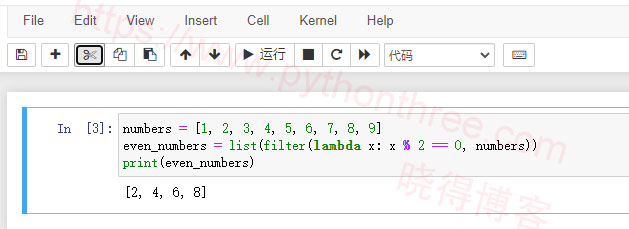
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers)
输出:
[2, 4, 6, 8]
3、使用map()函数应用转换
map() 函数可以与 lambda 函数结合使用,对列表中的每个元素应用转换。
示例:计算列表中每个数字的平方
numbers = [1, 2, 3, 4, 5]
squares = list(map(lambda x: x**2, numbers))
print(squares)
输出:
[1, 4, 9, 16, 25]
4、将 Lambda 函数与 functools.reduce() 结合使用
functools 模块中的 reduce() 函数可以与 lambda 函数结合使用,对列表中的元素累积应用二元运算,将列表缩减为单个值。
示例:计算列表中所有数字的乘积
from functools import reduce
numbers = [1, 2, 3, 4, 5]
product = reduce(lambda x, y: x * y, numbers)
print(product)
输出:
120
5、创建一次性的小型函数
Lambda 函数非常适合创建不需要正确函数定义的小型一次性函数。
示例:求两个数中的最大值
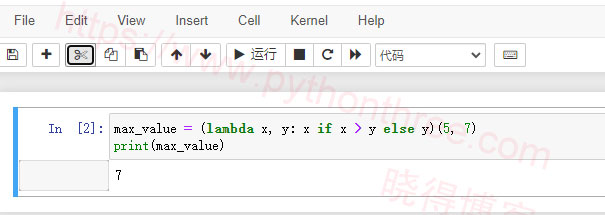
max_value = (lambda x, y: x if x > y else y)(5, 7)
print(max_value)
输出:
7
6、实现简单的事件处理程序
Lambda 函数可用于为用户界面元素(例如 GUI 应用程序中的按钮)创建简单的事件处理程序。
示例:在 Tkinter 应用程序中创建带有 lambda 函数的按钮
import tkinter as tk
def on_click():
print("Button clicked!")
root = tk.Tk()
button = tk.Button(root, text="Click me!", command=lambda: on_click())
button.pack()
root.mainloop()
7、在 Tkinter 的 GUI 编程中使用 Lambda 函数
Lambda 函数可用于为 Tkinter 小部件(例如按钮)创建简单的事件处理程序,而无需单独的函数。
示例:使用 lambda 函数创建一个按钮,单击时更新标签的文本
import tkinter as tk
def update_label(label):
label.config(text="Hello, World!")
root = tk.Tk()
label = tk.Label(root, text="Click the button!")
label.pack()
button = tk.Button(root, text="Click me!", command=lambda: update_label(label))
button.pack()
root.mainloop()
[python]
<h2 class="heading1">8. Working with Lambda Functions in pandas DataFrame Operations </h2>
Lambda functions can be used with the apply() and applymap() functions in pandas DataFrames to perform element-wise or row/column-wise operations.
Example: Apply a transformation to a pandas DataFrame column
[python]
import pandas as pd
data = {"A": [1, 2, 3], "B": [4, 5, 6]}
df = pd.DataFrame(data)
df["A"] = df["A"].apply(lambda x: x**2)
print(df)
输出:
A B
0 1 4
1 4 5
2 9 6
9、实现数据结构的自定义比较函数
Lambda 函数可用于为数据结构(例如 heapq 模块中的堆)创建自定义比较函数。
示例:根据数字的绝对值创建最小堆
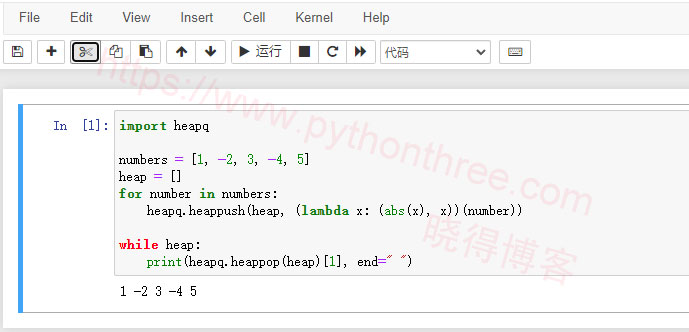
import heapq
numbers = [1, -2, 3, -4, 5]
heap = []
for number in numbers:
heapq.heappush(heap, (lambda x: (abs(x), x))(number))
while heap:
print(heapq.heappop(heap)[1], end=" ")
输出:
1 -2 3 -4 5
10、在 ThreadPoolExecutor 并发编程中使用 Lambda 函数
在进行并发编程时,例如使用并发.futures 模块中的 ThreadPoolExecutor 时,Lambda 函数可用于创建简单的一次性函数。
示例:使用 ThreadPoolExecutor 和 lambda 函数同时下载多个网页
import requests
from concurrent.futures import ThreadPoolExecutor
urls = ["https://www.example.com", "https://www.example.org", "https://www.example.net"]
results = []
with ThreadPoolExecutor() as executor:
futures = [executor.submit(lambda url: requests.get(url), url) for url in urls]
for future in futures:
results.append(future.result())
for result in results:
print(result.url, result.status_code)
结论
以上是晓得博客为你介绍的Python Lambda函数的10个实际用例的全部内容,Lambda 函数是 Python 中的一项多功能且强大的功能,使您能够为各种实际场景创建小型的一次性函数。希望通过在 Python 代码中理解并有效地使用 lambda 函数,可以使代码更加简洁、可读和高效。